Authentication
Everything there is to know.
โ๏ธ How it Works
We use the OAuth 2.0 flow for authentication, which is the industry standard for modern and secure APIs.
If you're already familiar with this authentication scheme from working on other APIs, a lot of these concepts will be easy to grasp for you - but if not, read on and we'll guide you through them.
Already a veteran?
There's a shorter page that just lists everything you need to know to authenticate.
Prerequisites
Before you continue, make sure that:
- If you're a library owner โ you've added the API module to your subscription, and you've generated a set of client credentials from the API Settings page.
- You don't have the API module yet? Reach out to your sales contact, or via [email protected].
- This also applies to IT partners of Apicbase customers.
- If you're a partner โ you already have access to a sandbox library and you've already generated your client credentials, or you have a set of credentials that was granted to you by the API team.
- Don't have access to Apicbase? Send us an introductory email via [email protected].
โ๏ธ Key Concepts
To start with, let's define a few concepts used in this page and throughout the documentation that relate to the OAuth flow:
- Application: this is any party that communicates with the Apicbase API. Every entity that sends requests is an application, this applies to partners as well as library owners. Library owners can of course gain access to their own data without requiring special authorization from another person, but they still need an application to access it.
- Client ID: this is a public ID that identifies an application. It is safe to share this ID and to expose it in client-side JavaScript applications.
- Client Secret: this is a confidential key that is specific to an application, serving as a form of password. It is imperative that this key is kept private and not shared with unauthorized individuals. Moreover, any requests involving this key should be transmitted via a secure (HTTPS) channel, and executed server-side. This key is used to generate access tokens and refresh them.
- Access Token: this is the bearer token included as a header in every request. It both tells Apicbase who you are, and allows us to determine whether you have access to the requested resource. This token is temporary and needs to be refreshed at least every 7 days.
- Refresh Token: this is a secondary token that does not need to be sent with every request. An application sends this token to Apicbase when it needs to generate a new access token, but more on this later.
- Authorization Code: this is a key with a very short lifespan, and is exclusively employed during the initial authentication process. Its purpose is to enable a user to authorize an application to access their data on their behalf.
These are the main players in the authentication process:
- Client: this is the party that interacts with the API, such as a partner application that utilizes Apicbase to support their back-end or an automated script that populates a local database with recipe images obtained from Apicbase. It's not an actual human, but rather an entity that interacts with the API programmatically!
- Resource owner: this is the real human that takes part in the authentication process. They possess ownership of the data within a library, and possess the power to authorize access to it. Whenever a client seeks to access a resource from Apicbase, Apicbase must ensure that the resource owner has granted authorization for the client to do so.
- Naturally, Apicbase also plays a role in the authentication process. As the host of the content owned by the resource owner, which the client seeks to access, we are responsible for ensuring that everyone is in agreement ๐
๐ The Authorization Code Flow
This does not apply to supplier integrations.
Integrated suppliers have their own authentication scheme, and the information in the rest of this page does not apply to that type of integration. Head over to the authentication for suppliers guide for details.
The OAuth 2.0 specification outlines various authentication flows that cater to different types of applications. For the Apicbase API, only the authorization code flow is supported. The following diagram outlines the steps involved in this flow:
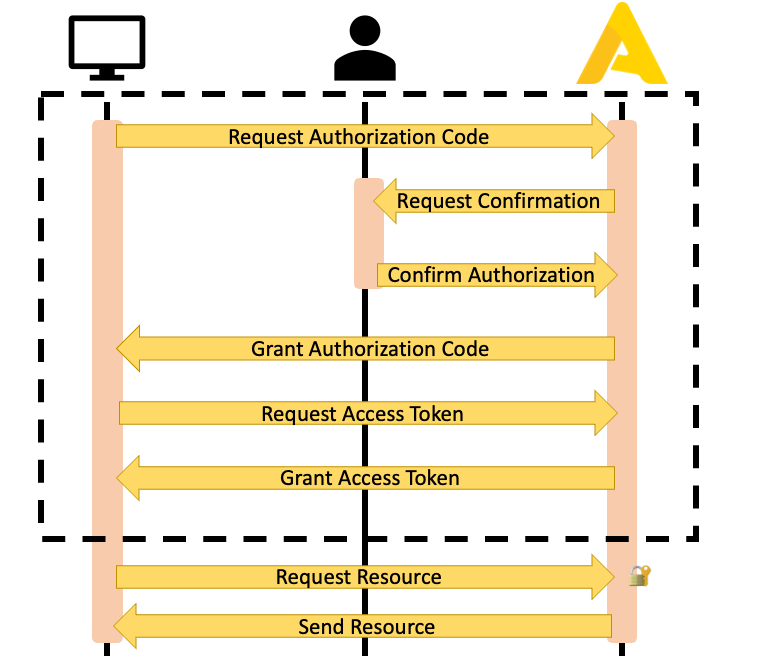
Request the Authorization Code
To begin the authorization process, your application must first direct the resource owner to the authorization page. This page will prompt them with a message asking if they allow the application to access the requested resources. The authorization URL takes the following form:
https://app.apicbase.com/oauth/authorize/?response_type=code&client_id=MY_CLIENT_ID&scope=SCOPE1+SCOPE2>
Replace MY_CLIENT_ID
with your application's Client ID and SCOPE1+SCOPE2
with the scopes that your application requires (for example, accounts+library
). Include a link to this URL from your application.
Users will be directed to an Apicbase login page where they will be prompted to enter their Apicbase credentials (email and password). Upon successful login, they will then be asked to confirm that they allow the application to access the requested resources through a confirmation screen.
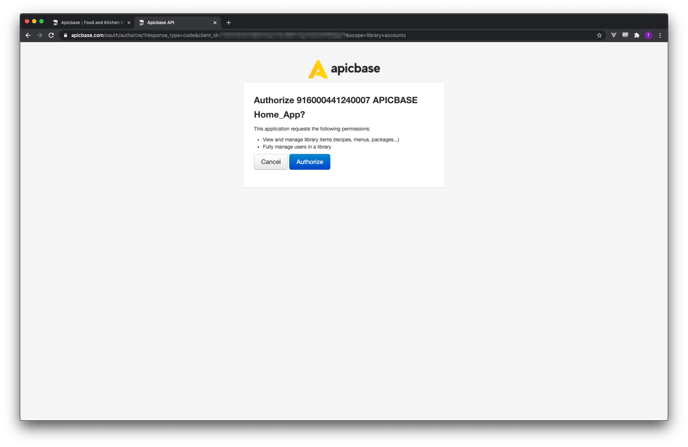
Once the user clicks 'Authorize', their browser window will be redirected to either your application's redirect address or an Apicbase page by default. The authorization code will be included in the URL as a query parameter, as shown below:
https://www.your-website.com/your-apicbase-subpage/?code=123456abcdef
You can host a script in this page to extract the authorization code from the URL.
The authorization code is only valid for one minute.
Exchange the Authorization Code for a Token
Once in possession of the authorization code, your application must exchange it for an access token. Make a POST request to the https://api.apicbase.com/oauth/token/
endpoint with the following payload:
grant_type=authorization_code
code=YOUR_AUTHORIZATION_CODE
redirect_uri=YOUR_REDIRECT
client_id=YOUR_CLIENT_ID
client_secret=YOUR_CLIENT_SECRET
This request must be encoded as x-www-form-urlencoded.
One alternative is to send the client credentials (client ID + client secret) in the Authorization header, encoded as Basic authentication. In this case, you can omit the client_id
and the client_secret
from the payload.
Perform this exchange only through secure channels.
The exchange involves confidential keys used to access customer data that should only be transmitted via secure channels (HTTPS). Do not perform this exchange using JavaScript or any other client-side methods, and do not under any circumstances deploy your client secret to a distributed app or a webpage. Only connect to Apicbase's authentication server via your back-end.
The authorization server will reply with an access token and a refresh token, as well as the allowed scopes and the access token's expiration time in seconds:
{
"access_token": "YOUR_ACCESS_TOKEN",
"expires_in": 604800,
"token_type": "Bearer",
"scope": "accounts library",
"refresh_token": "YOUR_REFRESH_TOKEN"
}
Did you get an error here?
No need to fret if you encounter an
invalid_grant
error during development! This error usually occurs when you try to exchange an expired authorization code for a token.Keep in mind that authorization codes are only valid for one minute, which can be a bit tight for humans. However, machines can usually complete the exchange within this time frame. If you do run out of time, don't worry - simply start over with a new authorization code and you'll be good to go!
๐ Making Requests with the Access Token
Congratulations, with the access token in your possession, you now have everything you need to start making API requests!
The access token is a bearer token. Include it in the Authorization header preceded by the string literal "Bearer ". Here's an example using cURL:
curl --request GET \
--url https://api.apicbase.com/api/v2/accounts/users \
--header 'accept: application/json' \
--header 'authorization: Bearer AEIOU12345'
๐ Using the Refresh Token
The access token has a lifespan of one week, which means it needs to be constantly refreshed. Every successful request to the /oauth/token/
endpoint also receives a refresh token as response, which can be used to request a new access token.
The request that produces a new token from the refresh token is very similar to the request that exchanges the authorization code for an initial set of tokens. Make a POST request to the https://api.apicbase.com/oauth/token/
endpoint with the following payload:
{
grant_type: "refresh_token",
client_id: "YOUR_CLIENT_ID",
client_secret: "YOUR_CLIENT_SECRET",
refresh_token: "MY_REFRESH_TOKEN"
}
Here, too, the content-type needs to be x-www-form-urlencoded.
To clarify, when the access token expires, your server can use the refresh token to obtain a new access token without requiring the user to re-authorize the application. This process is called refreshing the access token.
When refreshing the token, the authentication server will respond with a new access token, and a new refresh token that should be used for the subsequent requests. The old tokens are no longer valid, and should be discarded.
It's important to securely store the refresh token and only use it in a server-to-server request. Do not share or expose the refresh token to any client-side code or any third-party.
The refresh token has no fixed expiration date and remains valid even after the access token associated with it has expired. For instance, you may continue using the same access token until the API returns a 401 Unauthorized response (signifying that the token is no longer valid) and then refresh it using the refresh token.
Another error here?
Your application may also encounter an
invalid_grant
error during this step. If you receive this error while refreshing the access token, it indicates that the refresh token is not valid. This can happen when attempting to use a refresh token that has already been used.To prevent any issues, ensure that your application correctly discards used tokens and stores new refresh tokens. Additionally, it's recommended to keep separate sets of tokens for development and production environments. If a developer refreshes the same token used by the production application, the application will no longer be able to refresh its tokens!
๐ค User Permissions and the Active Library
This process will produce a user-based token for an application, which is bound to the same permissions as the user that you logged in with to generate it.
This also means that if that user switches libraries, the application will also start operating on a different library, sometimes unexpectedly. Read the guide on user-based tokens to understand how and why that happens, and to find ways to work around this.
๐ค Q&A
I'm stuck! Help?
You can refer to our quick start tutorial - it provides detailed step-by-step instructions that are specifically designed to guide first-time API users through the authentication process.
If you're really stuck, don't hesitate to reach out to our API experts at [email protected] for assistance!
I'm a customer and I want to access my own data via the API. Do I need this?
This information also applies to customers with IT partners who want to develop their own external projects using the Apicbase API.
Yes, customers accessing their own data follow the same authentication scheme as external third-parties. But if you have signed up for the API module, you can obtain your own client credentials from the API Settings page. These credentials should be used as the client credentials for the authentication process described on this page.
After following the steps manually once to obtain an initial set of tokens, you can set up a script to programmatically refresh the tokens for subsequent requests. You don't need to do it all over again every time, as long as your script correctly refreshes tokens!
I don't have a user-facing interface. Can I still integrate my app with Apicbase?
No problem at all! In this case, you can still obtain a set of client credentials from Apicbase. However, instead of directing your users to a login page to obtain an authorization code that you can exchange for an access token, you can simply ask them to add your application to the library and generate the access and refresh tokens for you. It's a little bit of overhead for the user but no less functional.
Direct your users to this article in our knowledge base, and ask them to copy the token details to you: How do I add a third-party application to my library?. Make sure to inform them properly of the scopes that your application requires.
Once your application is in possession of a set of tokens, it can interact normally with the Apicbase API. Note that as a partner, you need to request your application to be added to the dropdown selector in the API settings page, which we only do for approved partners. You still need to refresh these tokens properly!
Can I have an access token with a longer expiration date? Or one that doesn't expire?
No, this is not done because it compromises security. When developing for the Apicbase API, your application needs to be able to manage access tokens and refresh them correctly.
While it may seem a bit complicated at first, especially if you're not familiar with the authentication scheme, in the long run, this little bit of effort pays off and ensures that your application and the user's data are secure.
Can I have multiple access tokens at the same time?
Yes! This is a great idea if your application is distributed (such as a POS integration that connects to the Apicbase API from multiple physical installations) or to keep different credentials for development and production environments. Each set of tokens is independent, and refreshing one set does not invalidate the others.
What's the redirect URI?
If you implement the OAuth flow, you can optionally redefine this URL to have Apicbase redirect a user that logs in when producing an authorization code to a page hosted by your application. The default redirect is https://api.apicbase.com/auth-code/
, which is just a page that shows the authorization code embedded in the URL. If you host the login flow, you want the application to redirect to a page where some custom script will extract this code from the URL and exchange it for a token.
Redefining the redirect is not important if you're not implementing the user login flow. If you're a library owner or a partner with a sandbox account, you can do this straight from the API settings page. Partners with their own client credentials should email us at [email protected] if they need to redefine this URL.
This is kind of a lot. Why do you use OAuth?
OAuth is the industry-standard protocol for API authorization, widely adopted by the largest and most secure tech companies worldwide. It is so popular because it offers several advantages for both developers and users. It enables third-party applications to access user resources, but the developers (you!) don't need to worry about managing user passwords or the security risks that come with storing them.
Additionally, users can control which resources they allow the application to access and can revoke access at any time. OAuth also simplifies the authentication process for users, as they can often connect your solution to Apicbase with just a few clicks.
Lastly, it's an open and standardised protocol, which makes it easier for us to maintain and for developers to build for.
Updated 8 days ago
These topics are also important when you start developing for the Apicbase API: